Finding a framework
So, I have recently found myself with a need to dive into the depths of modern web-development and, as I am currently looking to write a highly interactive single-page web application, the first thing to decide on was a client side framework to use. You won't have to read too many of my blog posts to realise that I'm a big fan of Rx. I am also keen on the MVVM approach to separating concerns when writing user interfaces. Therefore, during my research of modern web UI frameworks, I was extremely interested when I came across WebRx .
Billed as "a browser-based MVVM-Framework that combines functional-reactive programming with declarative Data-Binding, Templating and Client-Side Routing" it struck a chord with my UI development style. In fact, it elevates Observables to a first class concept in a manner eerily similar to the ObservableProperty/ObservableCommand classes I wrote for my Caliburn.Micro.Reactive.Extensions package.
Unfortunately, as a framework, WebRx seems to be struggling to achieve critical mass and therefore there was very limited information available when I decided to attempt to implement the WebRx "Hello World" example using Visual Studio. As is almost par for the course when using a new framework, I fell at a frustratingly early hurdle and, given the lack of info (seriously, only one tagged post on stackoverflow!), I had to work through the problem 'old-skool'... ya'know, by actually finding and solving the problem rather than just googling a solution.
Anyway, I thought I'd put together a post outlining how to get started with this framework in Visual Studio in an attempt to start addressing the lack of info regarding this promising framework.
The name is Bart Simpson
Rather than the traditional 'Hello World' app, WebRx's 'Getting Started' guide displays a page stating 'The name is Bart Simpson'. This is done in order to demonstrates the MVVM separation of concerns through the use of a view bound to an underlying view model which provides the name 'Bart Simpson'.
I don't intend to cover the entirety of the getting started guide here, merely the additional/different steps needed to get the project work from Visual Studio. As such, I suggest opening the guide in a browser and leaving it open while working through the steps below as I will be referring to it extensively.
First up, open Visual Studio. Pretty much any modern version is fine, I am using Visual Studio 2015 Professional. Start a new project and select 'ASP.NET Web Application'
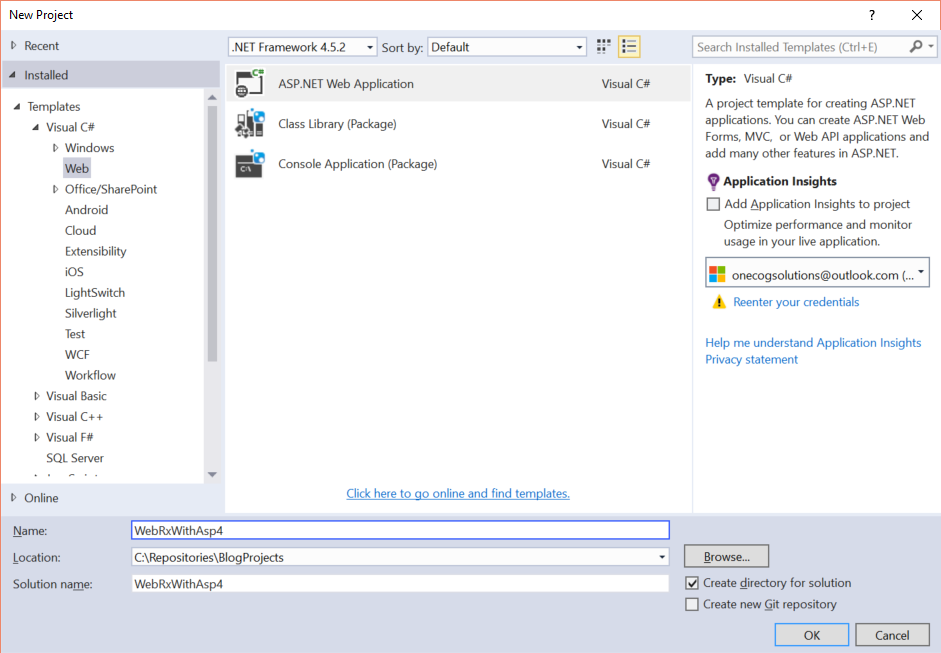
In the following dialog, select an empty ASP.NET Template and click ok.
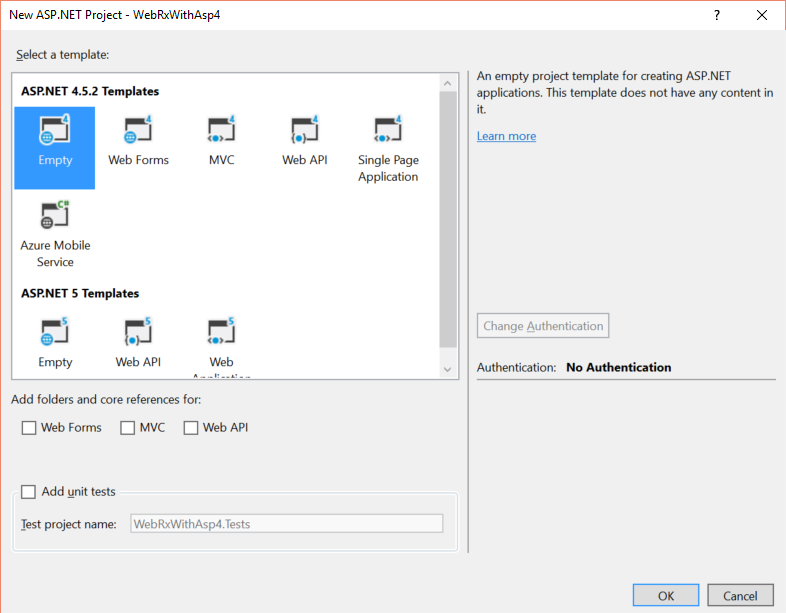
Now we have a web project, lets add a reference to WebRx. This can be done using either the Package Manager Console using the command Install-Package WebRx
or via the visual Nuget package manager as shown below:
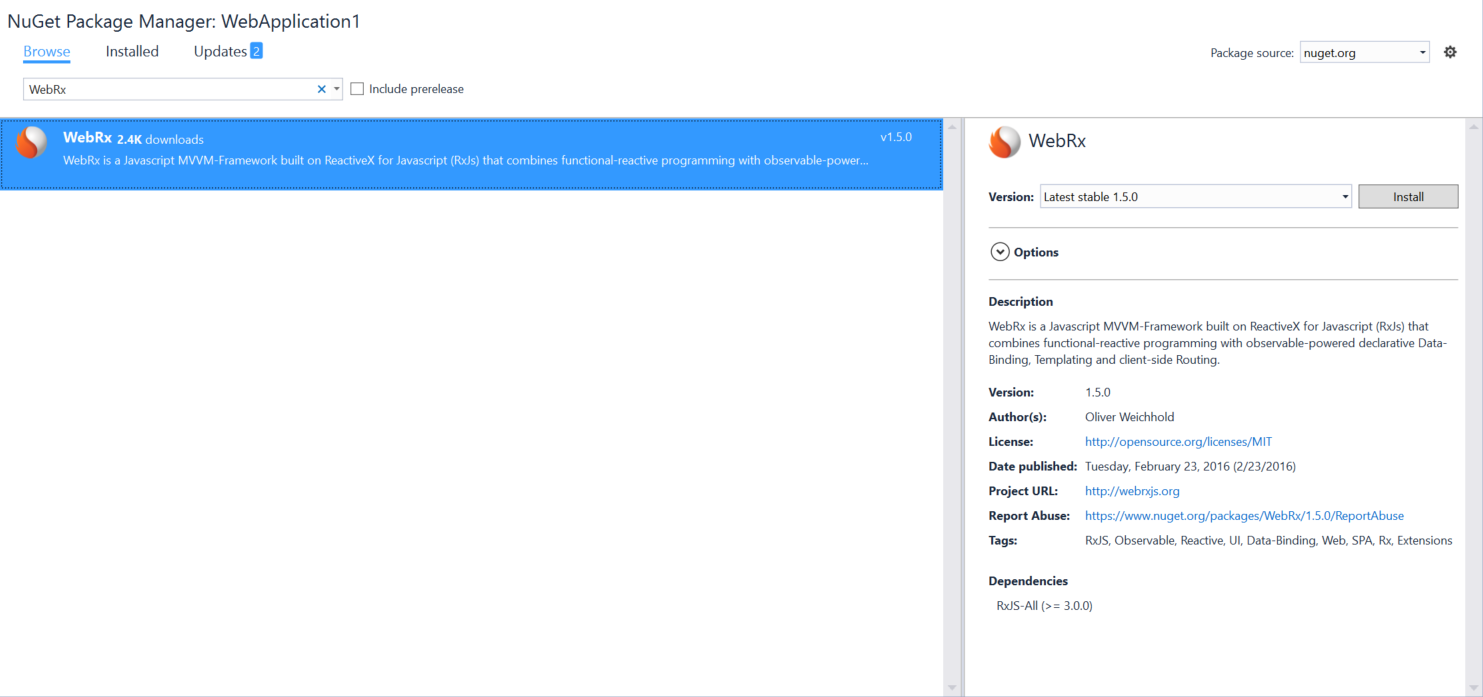
Regardless of how you add the reference to WebRx, you will be asked whether you wish to 'Search for TypeScript Typings' as shown below. Just click 'Yes'.
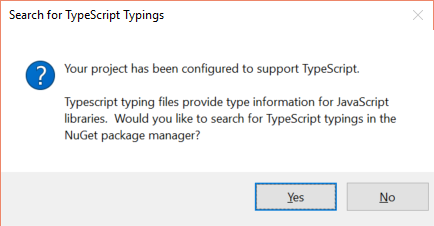
You will also be prompted to accept the license aggrement for a number of RxJs packages which WebRx depends upon; you should accept these too.
One the reference is added, you should find that a Scripts directory has been added to your solution and which contains a number of 'ts' and 'js' files for both WebRx and RxJs. With this in place, we can then continue with the getting started guide by adding an 'index.html' file to the project and copy pasting the sample 'index.html' file from the WebRx getting started guide. It should look something like this:
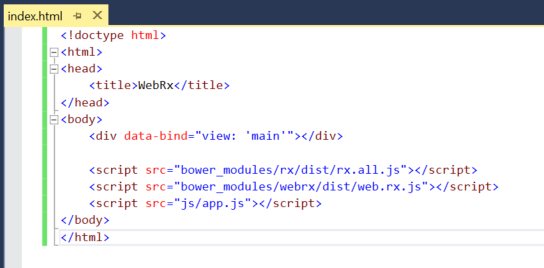
At this point the eagle-eyed amongst you will notice three things:
- Our scripts are now in a 'bower_modules' directory
As we used Nuget and not bower to install our WebRx dependencies, the script references should be changed to refer to the Scripts directory - Our scripts directory does not contain a rx.all.js file.
For some reason, WebRx depends on a version of RxJs that does not include an rx.all.js file. To resolve this, simply upgrade to the latest version of RxJs-All, as shown below: - We don't have a 'js' folder containing an 'app.js' file.
Because we've not got to that bit yet.
Now add a 'js' folder to the solution and add a 'app.js' to it. In this file copy the full 'app.js' sample from the WebRx getting started guide as shown below:
wx.app.component('hello', {
viewModel: function() {
this.firstName = 'Bart';
this.lastName = 'Simpson';
},
template: 'The name is <span data-bind="text: firstName + \' \' + lastName"></span>'
});
wx.router.state({
name: "$",
views: { 'main': "hello" }
});
wx.router.reload();
wx.applyBindings();
Save all, set 'index.html' as the start page and hit F5. If everything went as planned you should now see 'The name is Bart Simpson' displayed in your default browser:
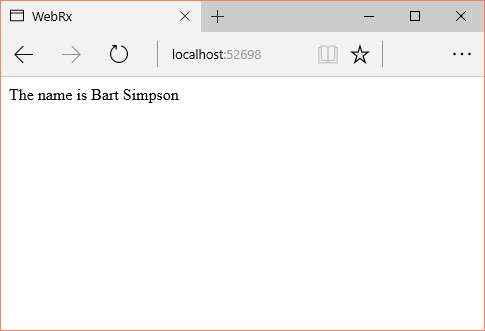
And that's it. While the getting started example doesn't seem very complex, it does show a separation of view and view model. I'm very much looking forward to digging into the details of this very promising framework.
The completed project for this post can be found in my BlogProjects repository on Github